Ant Design X - 快速构建AI对话应用的React组件库
5 min read
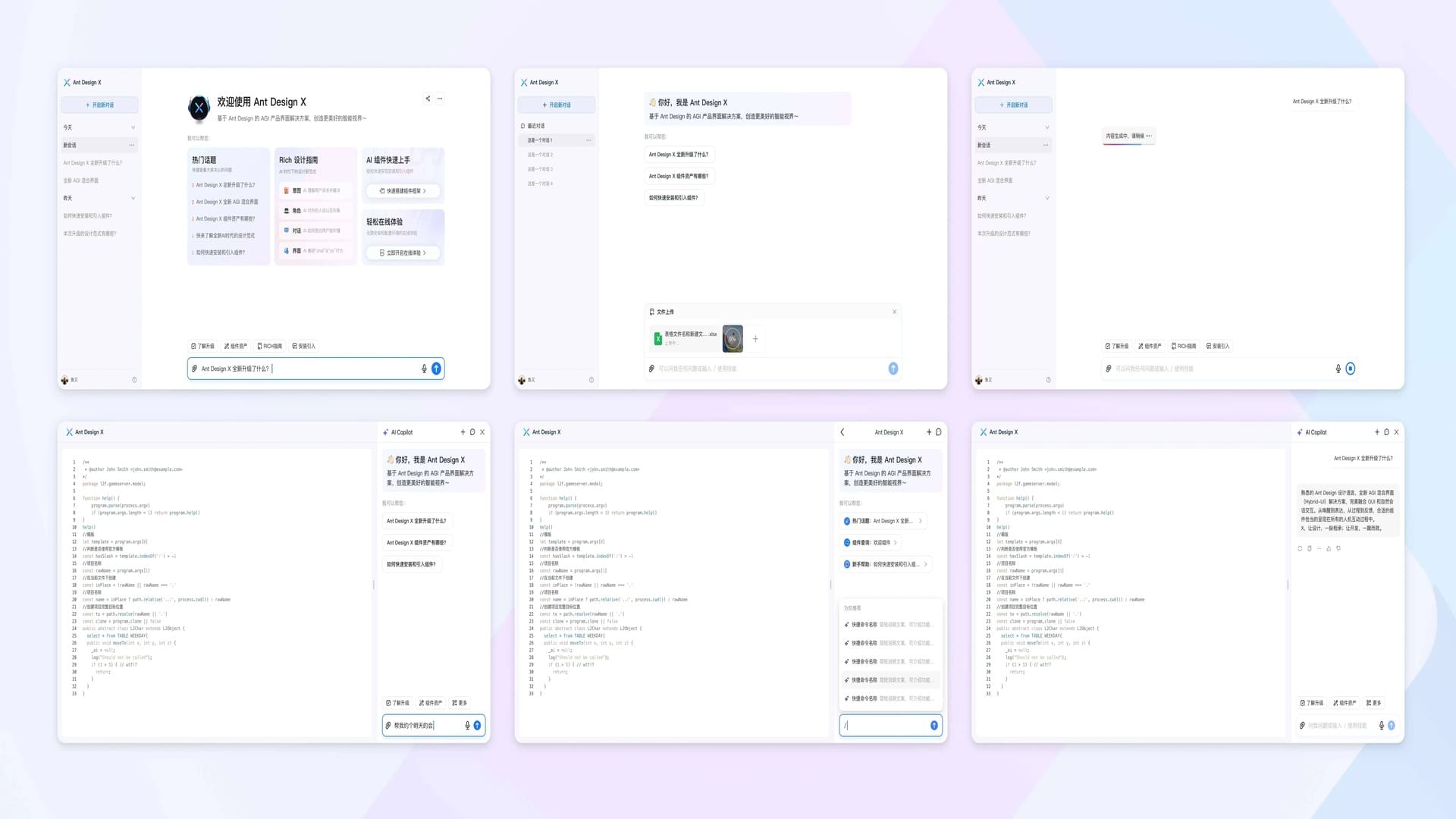
简介
Ant Design X
是一个专注于构建 AI 驱动的用户界面的 React 组件库。它提供了一套完整的解决方案,帮助开发者快速构建专业的 AI 交互界面。
核心特性
- 🌈 源自企业级 AI 产品的最佳实践:基于 RICH 交互范式,提供卓越的 AI 交互体验
- 🧩 灵活多样的原子组件:覆盖绝大部分 AI 对话场景,助力快速构建个性化 AI 交互页面
- ⚡ 开箱即用的模型对接能力:轻松对接符合 OpenAI 标准的模型推理服务
- 🔄 高效管理对话数据流:提供好用的数据流管理功能,让开发更高效
- 📦 丰富的样板间支持:提供多种模板,快速启动 LUI 应用开发
- 🛡 TypeScript 全覆盖:采用 TypeScript 开发,提供完整类型支持
- 🎨 深度主题定制能力:支持细粒度的样式调整,满足各种场景需求
安装使用
NPM 安装
# 使用 npm
npm install @ant-design/x --save
# 使用 yarn
yarn add @ant-design/x
# 使用 pnpm
pnpm add @ant-design/x
浏览器引入
你也可以使用 script 和 link 标签直接引入文件,并使用全局变量 antdx
:
<script src="https://unpkg.com/@ant-design/x/dist/antdx.min.js"></script>
注意: 强烈不推荐使用已构建文件,这样无法按需加载,而且难以获得底层依赖模块的 bug 快速修复支持。
重要:
antdx.js
和antdx.min.js
依赖react
、react-dom
、dayjs
,请确保提前引入这些文件。
基础使用示例
1. 简单的对话界面
import React from 'react';
import { Bubble, Sender } from '@ant-design/x';
const ChatDemo = () => {
const messages = [
{
content: 'Hello, Ant Design X!',
role: 'user',
}
];
return (
<div>
<Bubble.List items={messages} />
<Sender />
</div>
);
};
2. 对接模型服务
import { useXAgent, XRequest } from '@ant-design/x';
const { create } = XRequest({
baseURL: 'https://api.openai.com/v1',
dangerouslyApiKey: process.env['OPENAI_API_KEY'],
model: 'gpt-4',
});
const AIComponent = () => {
const [agent] = useXAgent({
request: async (info, callbacks) => {
const { message } = info;
const { onUpdate } = callbacks;
try {
create(
{
messages: [{ role: 'user', content: message }],
stream: true,
},
{
onUpdate: (chunk) => {
const content = JSON.parse(chunk.data).choices[0].delta.content;
onUpdate(content);
},
},
);
} catch (error) {
console.error(error);
}
},
});
return <Sender onSubmit={(msg) => agent.request({ message: msg })} />;
};
3. 完整的对话管理
import { useXAgent, useXChat, Bubble, Sender } from '@ant-design/x';
const ChatApp = () => {
const [agent] = useXAgent({
request: async (info, callbacks) => {
// 实现对话请求逻辑
},
});
const { messages, onRequest } = useXChat({ agent });
const items = messages.map(({ message, id }) => ({
key: id,
content: message,
}));
return (
<div>
<Bubble.List items={items} />
<Sender onSubmit={onRequest} />
</div>
);
};
按需加载
Ant Design X 默认支持基于 ES modules 的 tree shaking,这意味着你可以只引入需要使用的组件,未使用的组件代码不会打包到你的应用中:
import { Bubble } from '@ant-design/x'; // 只引入 Bubble 组件
TypeScript 支持
Ant Design X 使用 TypeScript 编写,提供完整的类型定义文件。要获得最佳的开发体验,建议使用 TypeScript 进行开发:
import type { BubbleProps } from '@ant-design/x';
const MyComponent: React.FC<BubbleProps> = (props) => {
// ...
};
最佳实践
- 按需引入组件:避免全量引入,减小打包体积
- 使用 TypeScript:获得更好的类型提示和开发体验
- 合理管理 API Key:不要在前端直接暴露敏感信息
- 实现错误处理:妥善处理 AI 服务可能的异常情况
- 优化用户体验:合理使用加载状态和反馈机制
总结
Ant Design X 为构建 AI 驱动的用户界面提供了一站式解决方案。通过其丰富的组件和便捷的开发体验,开发者可以快速构建出专业的 AI 对话应用。随着持续的更新和完善,它必将在推动智能交互发展方面发挥更大的作用。
更多文章
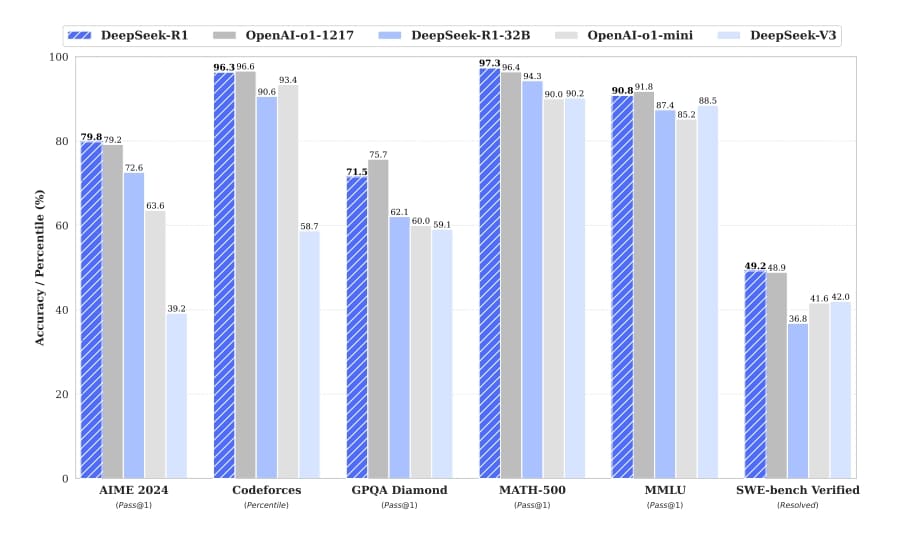
一文读懂 DeepSeek R1:强化学习如何重塑大语言模型推理能力?
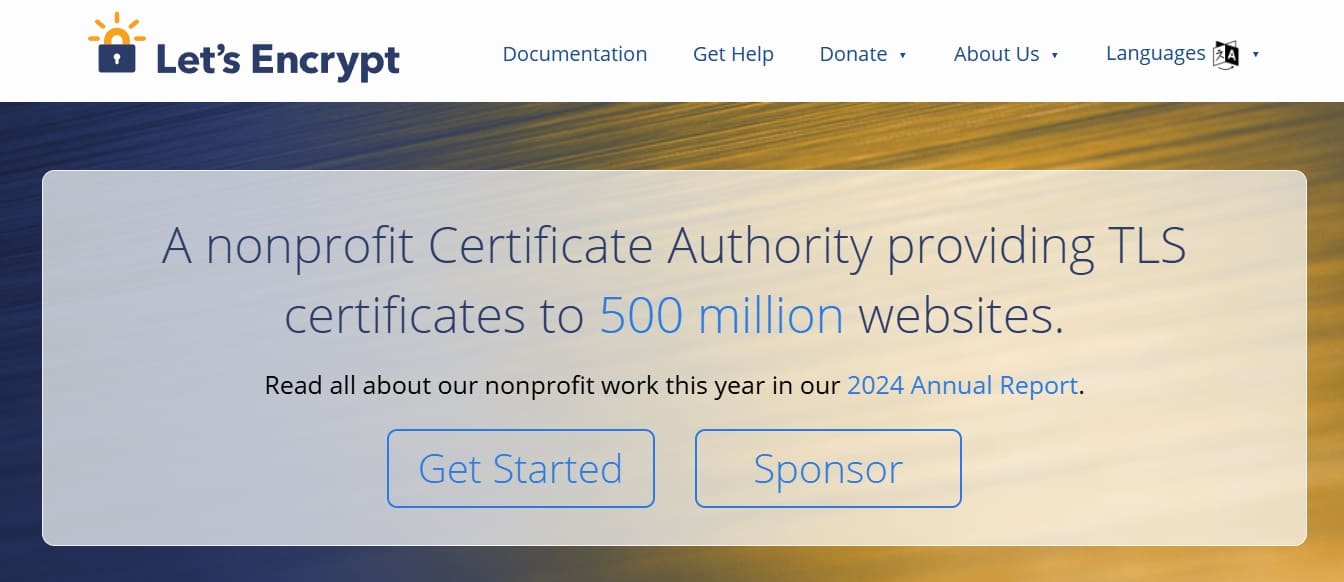
Let's Encrypt 将在2025年推出6天有效期证书和IP地址证书支持
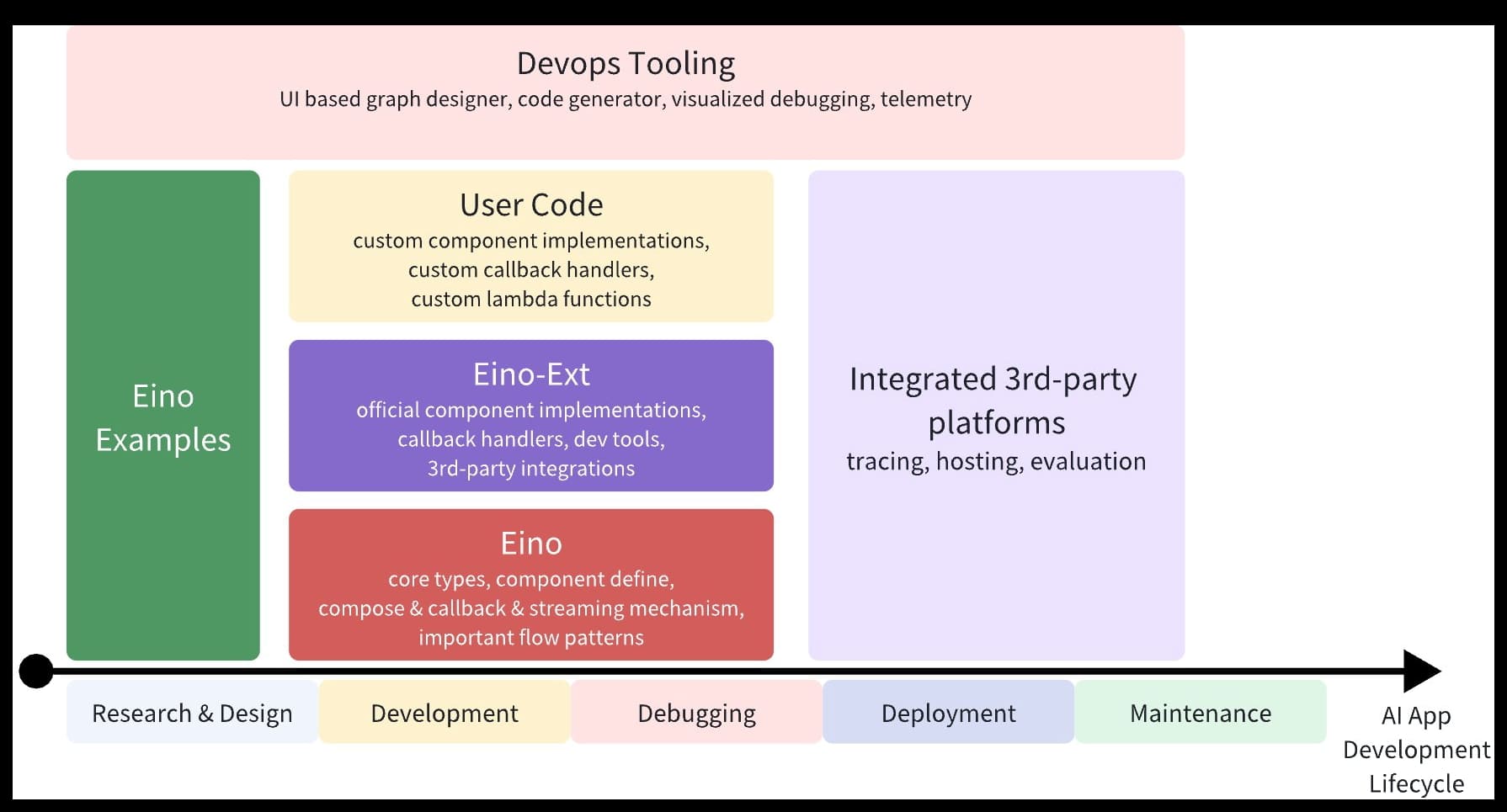
字节跳动开源基于 Golang 的大模型应用开发框架 Eino
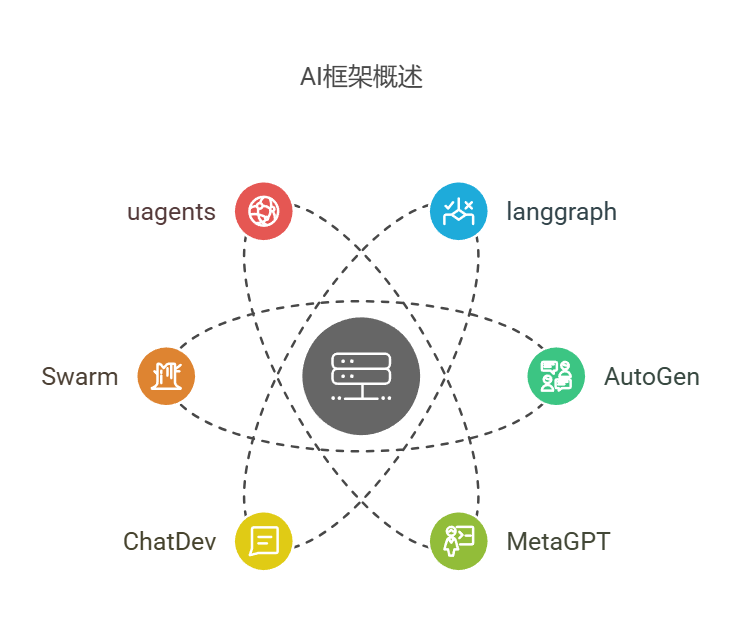
主流开源AI Agent框架对比与选型(langgraph, AutoGen, MetaGPT, ChatDev, Swarm, uagents)
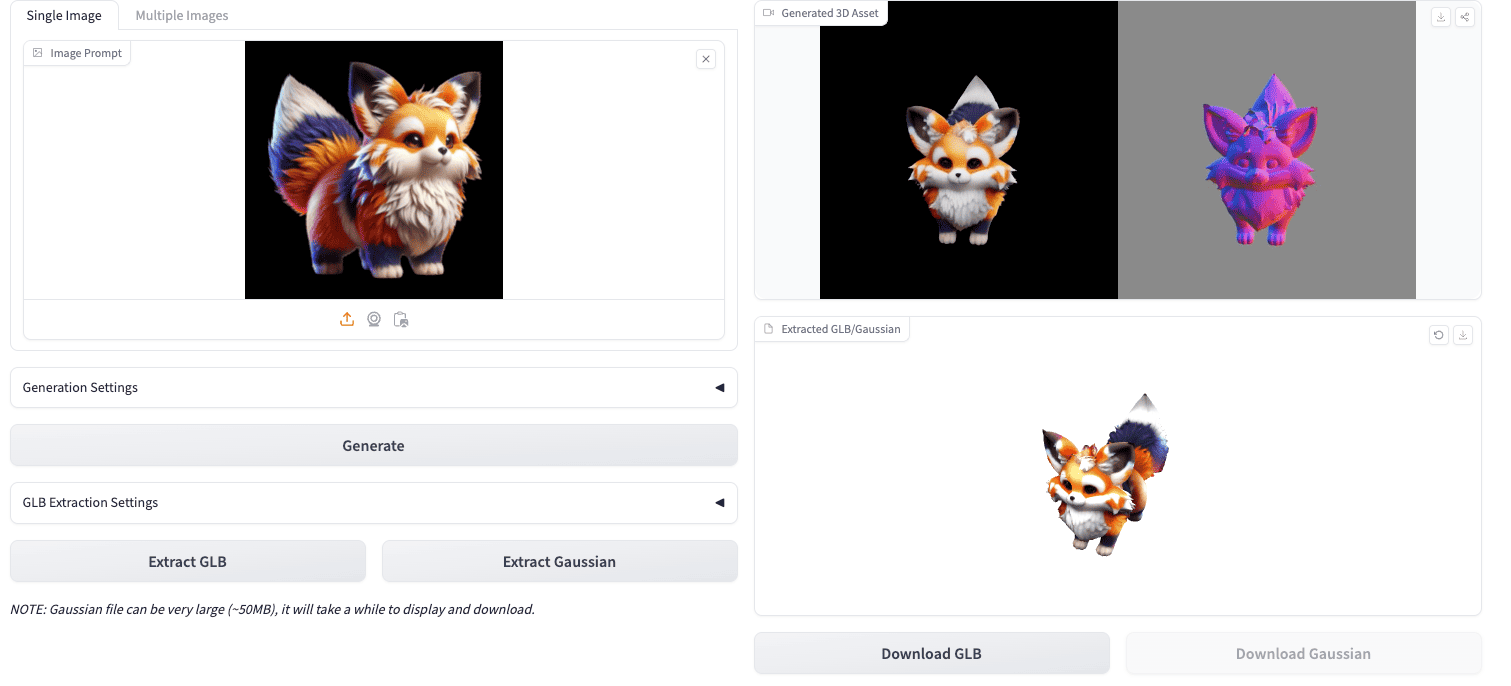
Microsoft TRELLIS 教程 - 开源文生3D、图生3D模型部署指南
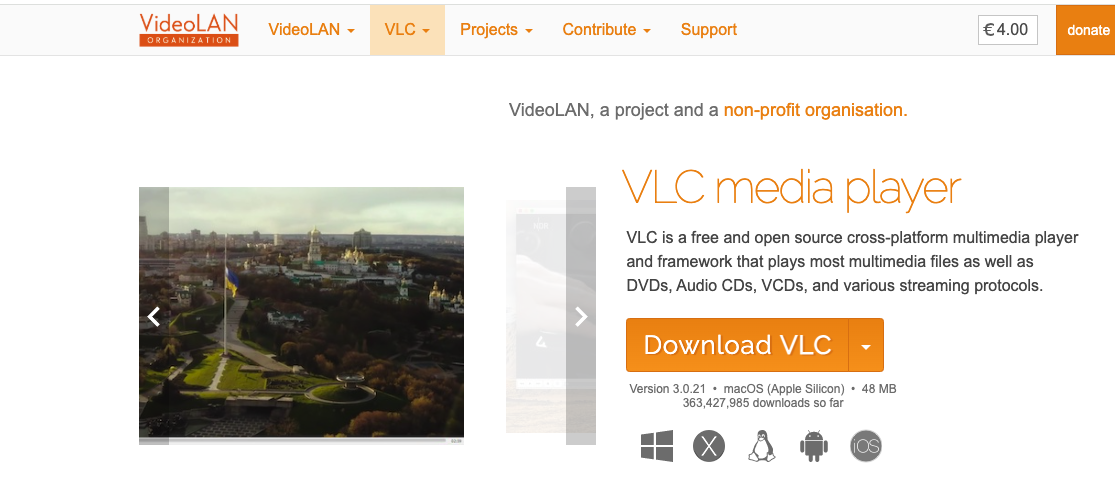
VLC 自动字幕和翻译(基于本地离线开源AI模型)|CES 2025
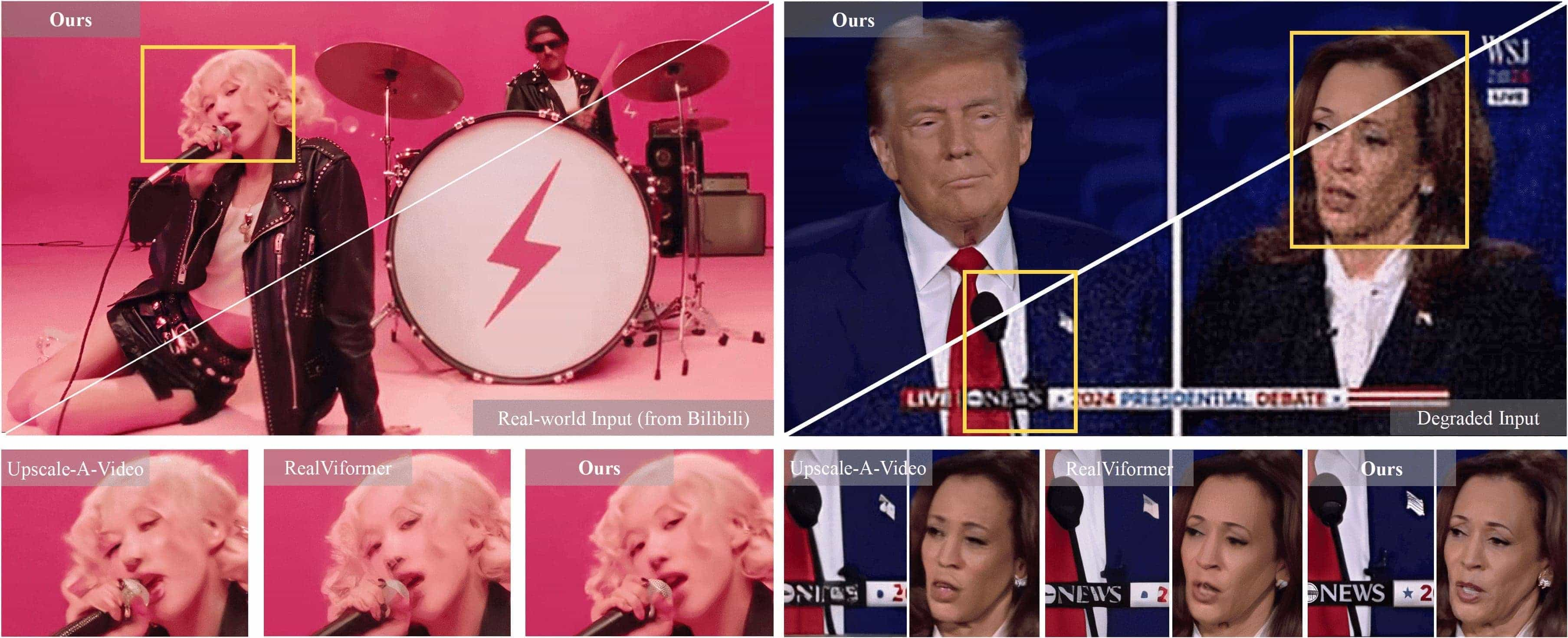
STAR: 基于文本到视频模型的实际场景视频超分辨率技术
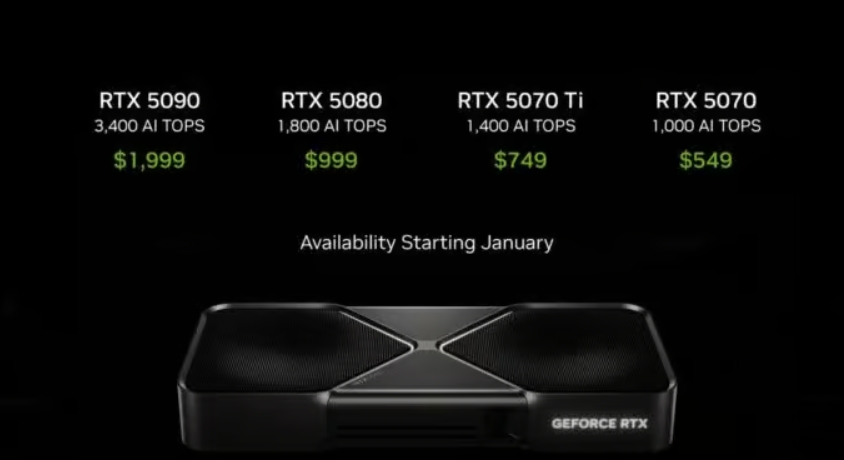
CES 2025 | 英伟达(NVIDIA) RTX 5090 震撼曝光 32GB GDDR7 显存开启显卡新纪元
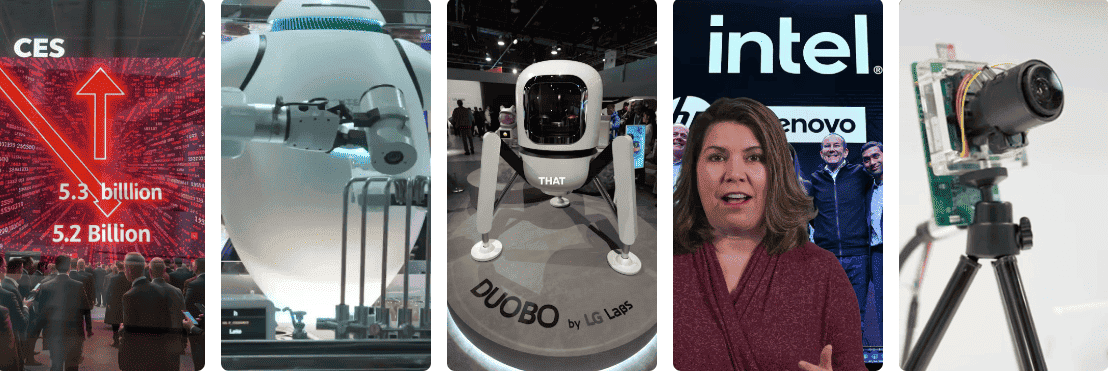
CES 2024 回顾:重温2024科技盛典精彩时刻
相关文章
暂无相关文章