Ant Design X - React Component Library for Building AI Chat Applications
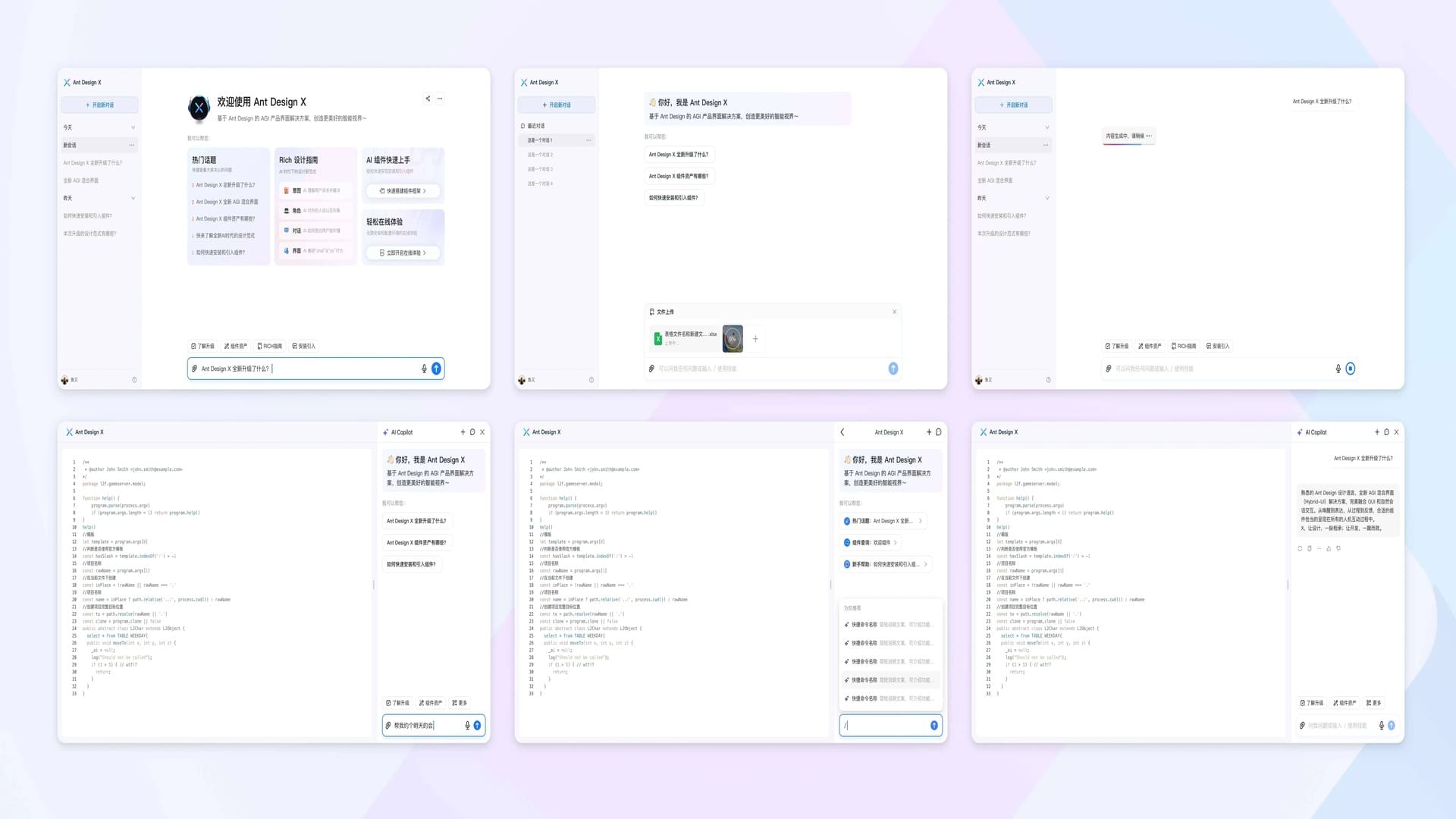
Introduction
Ant Design X
is a React component library focused on building AI-driven user interfaces. It provides a complete solution to help developers quickly build professional AI interaction interfaces.
Core Features
- 🌈 Best Practices from Enterprise AI Products: Based on RICH interaction paradigm, providing excellent AI interaction experience
- 🧩 Flexible Atomic Components: Covering most AI conversation scenarios, helping to quickly build personalized AI interaction pages
- ⚡ Out-of-the-box Model Integration: Easy integration with OpenAI-standard model inference services
- 🔄 Efficient Conversation Data Flow Management: Providing useful data flow management features for more efficient development
- 📦 Rich Template Support: Providing multiple templates to quickly start LUI application development
- 🛡 Full TypeScript Coverage: Developed with TypeScript, providing complete type support
- 🎨 Deep Theme Customization: Supporting fine-grained style adjustments to meet various scenario needs
Installation
NPM Installation
# Using npm
npm install @ant-design/x --save
# Using yarn
yarn add @ant-design/x
# Using pnpm
pnpm add @ant-design/x
Browser Import
You can also directly include the files using script and link tags, and use the global variable antdx
:
<script src="https://unpkg.com/@ant-design/x/dist/antdx.min.js"></script>
Note: Using pre-built files is strongly discouraged as it prevents tree-shaking and makes it difficult to receive quick bug fixes for underlying dependency modules.
Important:
antdx.js
andantdx.min.js
depend onreact
,react-dom
, anddayjs
. Please ensure these files are included beforehand.
Basic Usage Examples
1. Simple Chat Interface
import React from 'react';
import { Bubble, Sender } from '@ant-design/x';
const ChatDemo = () => {
const messages = [
{
content: 'Hello, Ant Design X!',
role: 'user',
}
];
return (
<div>
<Bubble.List items={messages} />
<Sender />
</div>
);
};
2. Model Service Integration
import { useXAgent, XRequest } from '@ant-design/x';
const { create } = XRequest({
baseURL: 'https://api.openai.com/v1',
dangerouslyApiKey: process.env['OPENAI_API_KEY'],
model: 'gpt-4',
});
const AIComponent = () => {
const [agent] = useXAgent({
request: async (info, callbacks) => {
const { message } = info;
const { onUpdate } = callbacks;
try {
create(
{
messages: [{ role: 'user', content: message }],
stream: true,
},
{
onUpdate: (chunk) => {
const content = JSON.parse(chunk.data).choices[0].delta.content;
onUpdate(content);
},
},
);
} catch (error) {
console.error(error);
}
},
});
return <Sender onSubmit={(msg) => agent.request({ message: msg })} />;
};
3. Complete Conversation Management
import { useXAgent, useXChat, Bubble, Sender } from '@ant-design/x';
const ChatApp = () => {
const [agent] = useXAgent({
request: async (info, callbacks) => {
// Implement conversation request logic
},
});
const { messages, onRequest } = useXChat({ agent });
const items = messages.map(({ message, id }) => ({
key: id,
content: message,
}));
return (
<div>
<Bubble.List items={items} />
<Sender onSubmit={onRequest} />
</div>
);
};
Tree Shaking
Ant Design X supports ES modules-based tree shaking by default, meaning you can import only the components you need, and unused component code won’t be bundled into your application:
import { Bubble } from '@ant-design/x'; // Only import the Bubble component
TypeScript Support
Ant Design X is written in TypeScript and provides complete type definition files. For the best development experience, it’s recommended to use TypeScript:
import type { BubbleProps } from '@ant-design/x';
const MyComponent: React.FC<BubbleProps> = (props) => {
// ...
};
Best Practices
- Import Components as Needed: Avoid importing everything to reduce bundle size
- Use TypeScript: Get better type hints and development experience
- Properly Manage API Keys: Don’t expose sensitive information in the frontend
- Implement Error Handling: Properly handle potential AI service exceptions
- Optimize User Experience: Use loading states and feedback mechanisms appropriately
Summary
Ant Design X provides a one-stop solution for building AI-driven user interfaces. Through its rich components and convenient development experience, developers can quickly build professional AI chat applications. With continuous updates and improvements, it will play an increasingly important role in advancing intelligent interaction development.
More Articles
![OpenAI 12-Day Technical Livestream Highlights Detailed Report [December 2024]](/_astro/openai-12day.C2KzT-7l_1ndTgg.jpg)
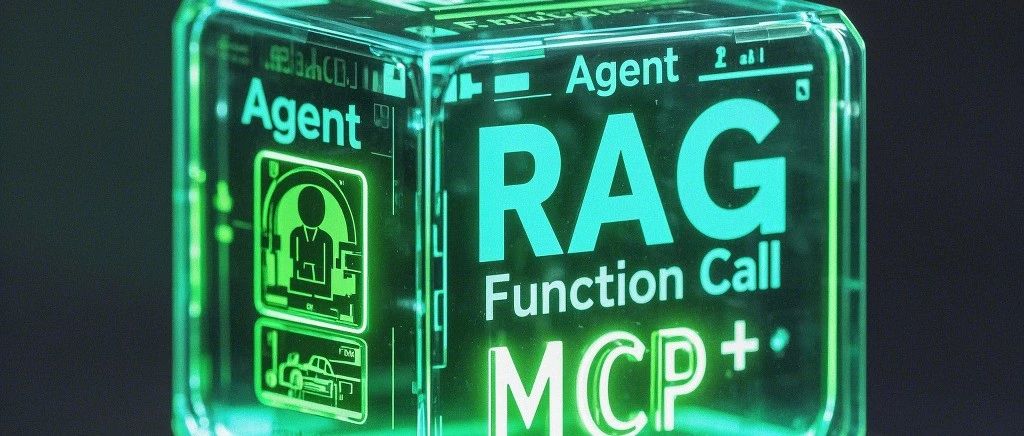
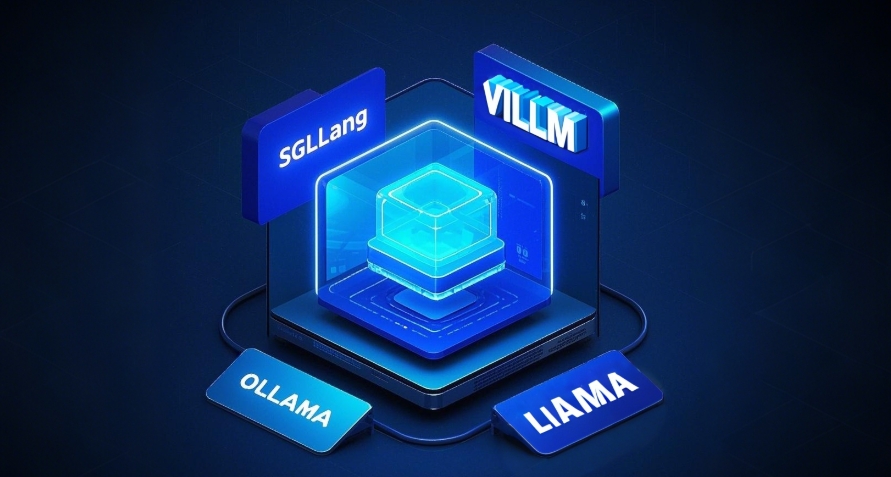
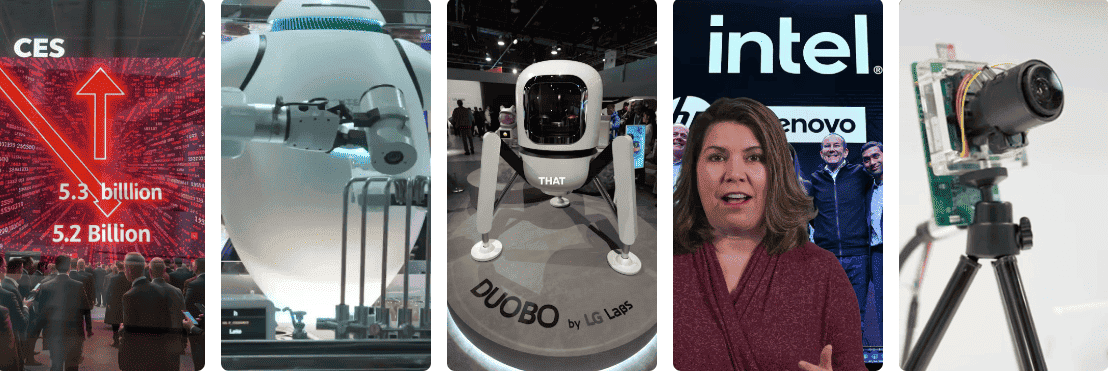
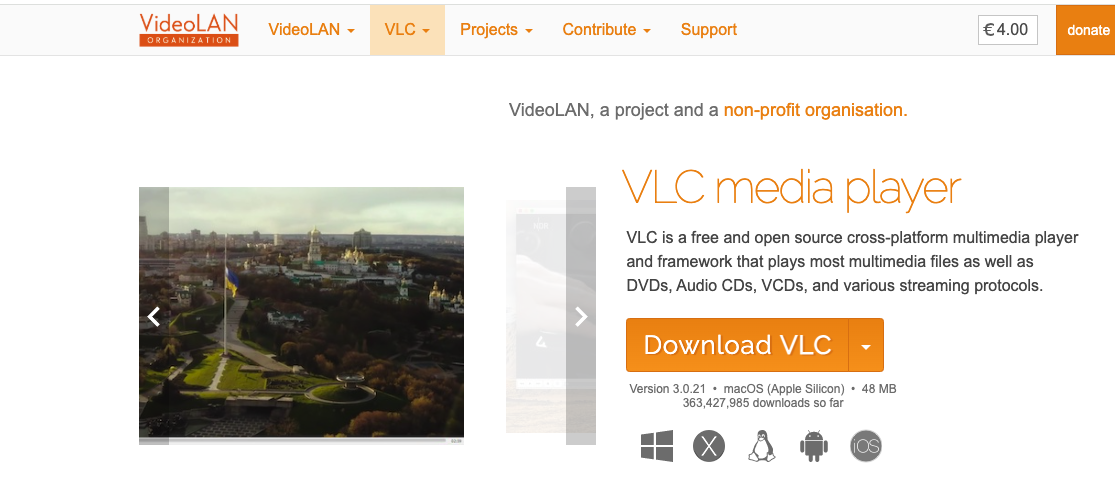
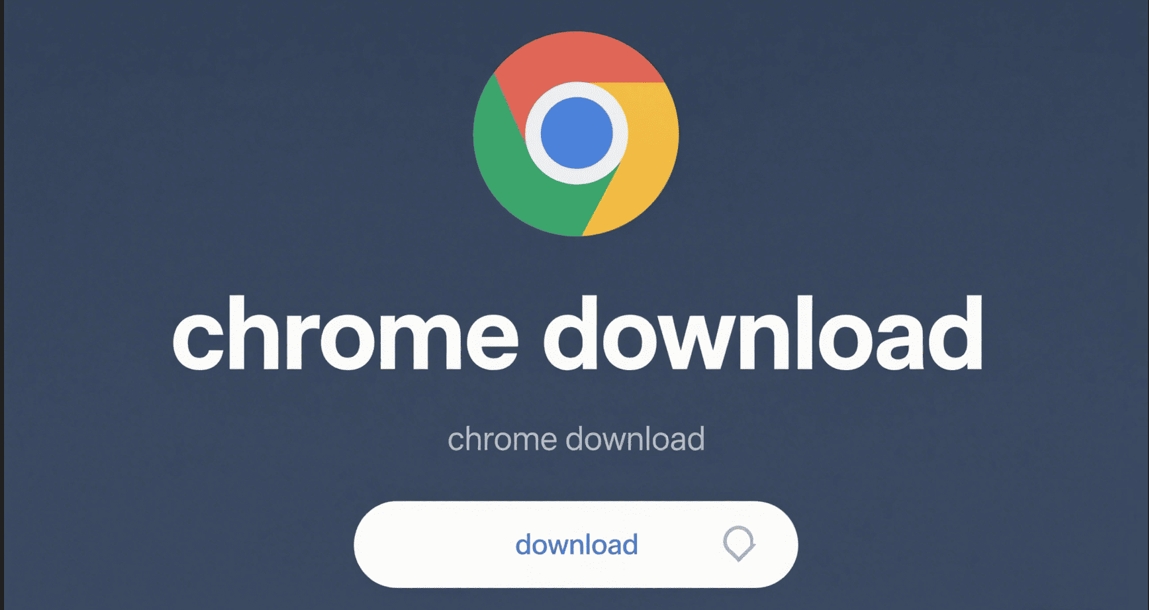
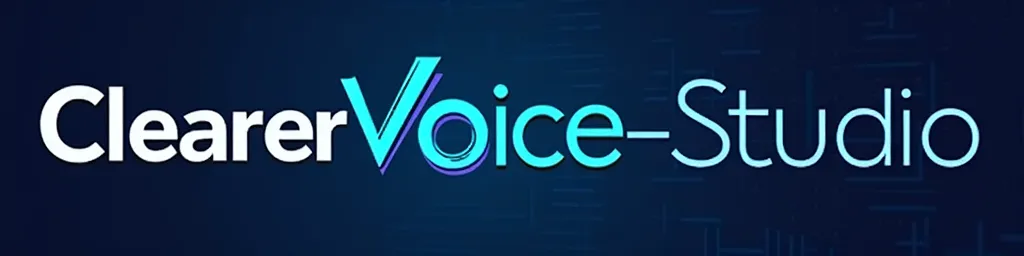
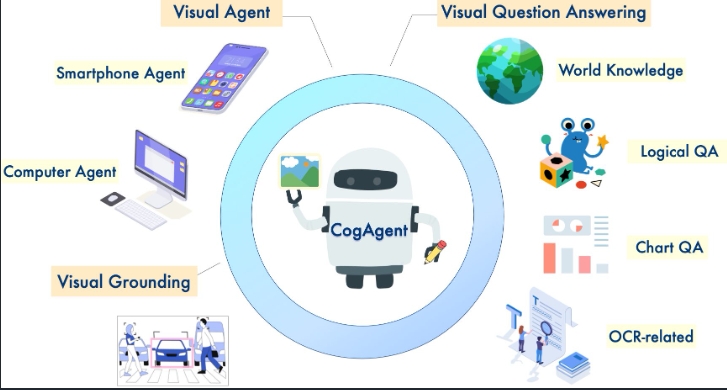
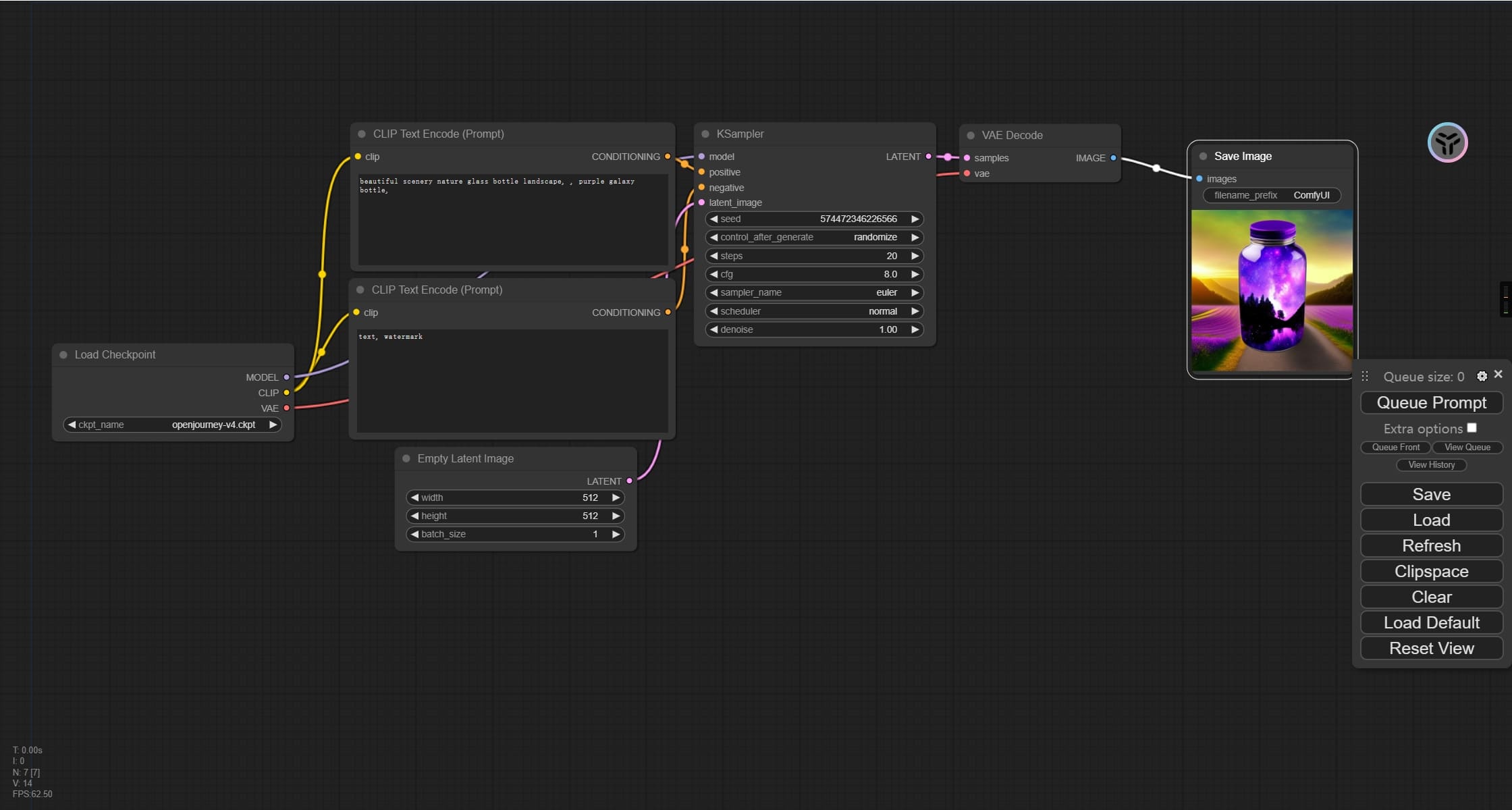
Related Posts
No related posts yet